Category: WooCommerce
What are WooCommerce endpoints?
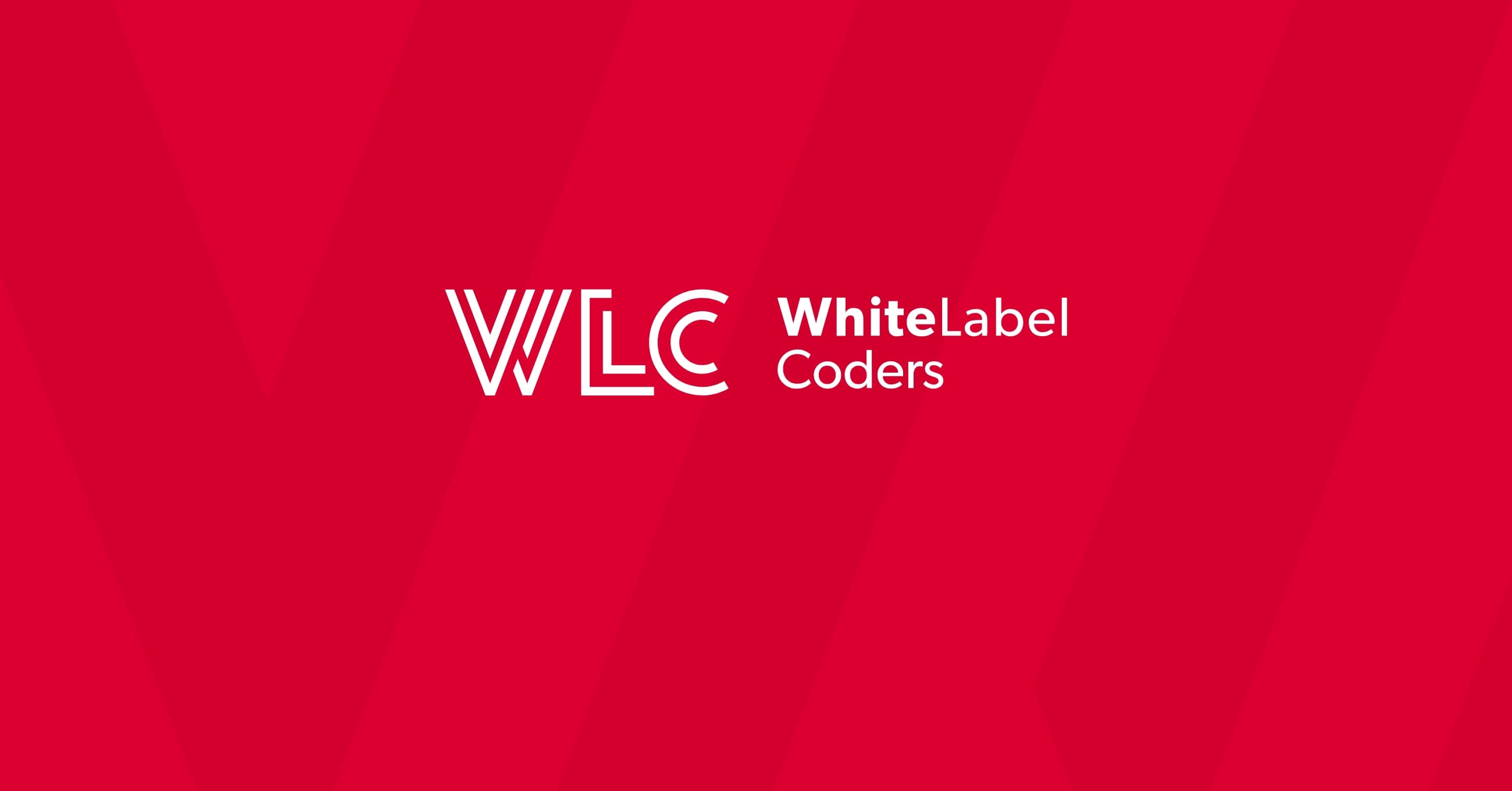
Ever wondered how your WooCommerce store knows where to send customers after they log in or check out? Or how your store’s data becomes available to mobile apps and other services? The magic behind these capabilities lies in a powerful but often overlooked feature: WooCommerce endpoints. Whether you’re managing a small boutique shop or developing a sophisticated e-commerce ecosystem, understanding endpoints can transform your approach to WooCommerce development.
In this comprehensive guide, we’ll demystify WooCommerce endpoints, explain their critical role in modern e-commerce applications, and show you how to leverage them for more flexible, powerful online stores. Ready to take your WooCommerce knowledge to the next level?
Understanding WooCommerce endpoints: A comprehensive introduction
Before diving into the technical details, let’s clarify what endpoints actually are. In the context of web development, an endpoint is simply a specific URL that represents a function or resource within your application. Think of endpoints as doorways that allow access to different rooms in your e-commerce house.
For WooCommerce specifically, endpoints serve two primary purposes:
- They define customer-facing URLs for account pages, checkout processes, and other user interactions
- They provide access points for programmatic data exchange through the REST API
Why should you care about endpoints? Because they’re the foundation of how your WooCommerce store interacts with both users and external systems. Mastering endpoints allows you to:
- Customize user journeys through your store
- Build headless commerce solutions
- Integrate with mobile apps and third-party services
- Create custom functionality beyond standard WooCommerce features
As e-commerce grows increasingly interconnected, the ability to skillfully work with endpoints becomes not just useful but essential for competitive store development.
What are WooCommerce REST API endpoints?
The WooCommerce REST API is a powerful interface that allows developers to interact with store data programmatically. REST (Representational State Transfer) is an architectural style for building APIs that’s based on standard HTTP methods.
WooCommerce REST API endpoints are specific URLs that follow a consistent pattern:
/wp-json/wc/v3/[resource]
Where [resource]
represents the type of data you’re trying to access—products, orders, customers, etc.
These endpoints allow developers to:
- Retrieve store data (GET requests)
- Create new resources like products or orders (POST requests)
- Update existing resources (PUT requests)
- Delete resources (DELETE requests)
The true power of the REST API becomes apparent in headless commerce scenarios, where the front-end presentation layer is separate from the back-end WooCommerce system. This architectural approach enables the creation of custom shopping experiences across websites, mobile apps, and other digital touchpoints, all powered by the same WooCommerce core.
The WooCommerce REST API doesn’t just allow data access—it opens up entirely new possibilities for how your store can operate and integrate with the broader digital ecosystem.
Core WooCommerce endpoints and their functions
WooCommerce includes a range of built-in endpoints out of the box. These can be divided into two main categories: customer-facing URL endpoints and REST API endpoints.
Endpoint Type | Examples | Function |
---|---|---|
Customer Account Endpoints | orders, downloads, edit-address | Control URLs for customer account pages |
Checkout Process Endpoints | checkout, cart, thank-you | Manage shopping and checkout flow |
Product API Endpoints | /wp-json/wc/v3/products | Manage product data |
Order API Endpoints | /wp-json/wc/v3/orders | Process and manage orders |
Customer API Endpoints | /wp-json/wc/v3/customers | Customer data management |
Each of these endpoint categories plays a crucial role in the overall functionality of your WooCommerce store. For instance, the product endpoints allow developers to programmatically create, read, update, and delete products, while order endpoints enable sophisticated order management workflows.
Understanding these core endpoints provides the foundation for both customizing the standard WooCommerce experience and extending it with custom functionality. For store owners looking to scale their WooCommerce development capabilities, mastering these endpoints is a worthwhile investment.
How do WooCommerce account endpoints work?
Account endpoints are the URLs that control what customers see when they navigate to different sections within their account area. By default, WooCommerce registers several account endpoints:
- Dashboard: The main account page
- Orders: Customer order history
- Downloads: Downloadable products
- Addresses: Shipping and billing addresses
- Payment methods: Saved payment information
- Account details: Personal information
- Logout: End the current session
These endpoints work through WordPress’s rewrite rules system. When a customer visits, for example, /my-account/orders/
, WooCommerce intercepts this request and loads the appropriate content for displaying orders.
What makes account endpoints particularly interesting is that they can be customized. You can:
- Change the URL structure (e.g., “purchase-history” instead of “orders”)
- Add entirely new account sections
- Remove default sections you don’t need
- Rearrange the order of sections in the account menu
This flexibility allows you to create tailored customer experiences that match your specific business requirements. For instance, a subscription-based business might add a custom “Subscriptions” endpoint, while a B2B store might add a “Company Information” endpoint.
Creating custom WooCommerce endpoints
One of the most powerful aspects of WooCommerce’s architecture is the ability to create your own custom endpoints. This allows you to extend functionality beyond what’s available out-of-the-box.
Here’s a basic example of how to add a custom account endpoint:
// Register the endpoint function custom_add_my_account_endpoint() { add_rewrite_endpoint( 'my-custom-endpoint', EP_ROOT | EP_PAGES ); } add_action( 'init', 'custom_add_my_account_endpoint' ); // Add new menu item in account navigation function custom_add_my_account_menu_item( $items ) { $items['my-custom-endpoint'] = 'My Custom Page'; return $items; } add_filter( 'woocommerce_account_menu_items', 'custom_add_my_account_menu_item' ); // Add content to the custom endpoint function custom_my_account_endpoint_content() { echo '<h3>My Custom Content</h3>'; echo '<p>Here is the content for my custom account section.</p>'; } add_action( 'woocommerce_account_my-custom-endpoint_endpoint', 'custom_my_account_endpoint_content' ); // Don't forget to flush rewrite rules function custom_flush_rewrite_rules() { flush_rewrite_rules(); } register_activation_hook( __FILE__, 'custom_flush_rewrite_rules' );
This basic pattern can be adapted to create sophisticated custom functionality within the account area. For more complex requirements, you might want to work with a WooCommerce development specialist who can ensure that your custom endpoints are implemented securely and efficiently.
Creating custom REST API endpoints
Similarly, you can create custom REST API endpoints to expose new functionality programmatically:
add_action( 'rest_api_init', function () { register_rest_route( 'my-plugin/v1', '/custom-data', array( 'methods' => 'GET', 'callback' => 'my_custom_endpoint_handler', 'permission_callback' => function() { return current_user_can( 'manage_options' ); } )); }); function my_custom_endpoint_handler( $request ) { // Process the request and return data $data = array( 'status' => 'success', 'message' => 'Custom data retrieved' ); return new WP_REST_Response( $data, 200 ); }
This approach allows you to extend WooCommerce’s capabilities in virtually limitless ways, from creating custom reporting systems to building integrations with external services.
Security best practices for WooCommerce endpoint development
When working with WooCommerce endpoints, security should be a top priority. Here are essential practices to follow:
Security Concern | Best Practice | Implementation Approach |
---|---|---|
Authentication | Always verify user identity before allowing access to sensitive data | Use WooCommerce’s built-in authentication methods or implement OAuth for API access |
Authorization | Check that authenticated users have appropriate permissions | Implement proper capability checks (e.g., current_user_can()) |
Data Validation | Never trust input data from users or external systems | Validate and sanitize all input using WordPress functions like sanitize_text_field() |
Rate Limiting | Prevent abuse through excessive API calls | Implement request throttling for API endpoints |
Error Handling | Don’t expose sensitive information in error messages | Use generic error messages in production environments |
For API endpoints specifically, consider implementing an API key system or OAuth authentication to ensure that only authorized applications can access your store’s data. WooCommerce includes built-in support for API keys that can be generated and managed through the admin interface.
Remember that endpoints can expose sensitive customer and order data, so proper security measures are not optional—they’re essential for maintaining customer trust and complying with data protection regulations.
Key takeaways: Mastering WooCommerce endpoints for improved development
As we’ve explored throughout this guide, WooCommerce endpoints are fundamental building blocks for creating flexible, powerful e-commerce experiences. Here’s what you should remember:
- Endpoints serve both customer-facing navigation and programmatic API access
- Understanding endpoint architecture allows you to customize the customer experience
- Custom endpoints enable you to extend WooCommerce beyond its out-of-the-box capabilities
- Security is paramount when working with endpoints, especially those exposing sensitive data
- The REST API opens up possibilities for headless commerce and integrated experiences
For those looking to take their WooCommerce stores to the next level, endpoints provide the architectural framework to build truly custom solutions. Whether you’re looking to streamline the customer account area, develop mobile app integrations, or create unique shopping experiences, mastering endpoints gives you the tools you need.
Ready to put this knowledge into practice? Start by reviewing your current WooCommerce setup and identifying opportunities where custom endpoints could improve your customer experience or business operations. For complex implementations, consider partnering with experienced WooCommerce development specialists who can help you implement these powerful features securely and effectively.
Have you worked with WooCommerce endpoints before? What custom functionality have you implemented using them? The possibilities are virtually endless—limited only by your imagination and business requirements.